Need to spend some time crafting thought pieces and connecting with entrepreneurs and experts on LinkedIn? Will it benefit your or your customer’s business? Or does it just increase the vanity metric?
This dilemma isn’t uncommon, but research has an answer. You can further allay any lingering doubts by using LinkedIn’s application programming interface (API) to optimize your content distribution. Learn how to post to your company page or user page using the LinkedIn API.
Why LinkedIn is so important to your business
LinkedIn is the preferred hub for B2B marketing and sales. B2B Marketing Survey Experts show that LinkedIn is effectively leading all other social networks. About 77% said they got the best results on the free platform, while 79% on the paid platform agreed, well ahead of other platforms.
LinkedIn users seem to agree. Many people associate LinkedIn with resumes and jobs, but in reality You get 15x impressions for your content. for job postings. Other surveys 75% of potential buyers rate vendors based on thought leadership, and 70% of users view LinkedIn as a trusted source.
Clearly, increased content distribution on LinkedIn will give you an excellent return on your time invested. Businesses can increase these returns by using APIs and automation to scale their LinkedIn marketing while reducing the effort of doing everything manually.
Introducing the LinkedIn API Environment
The LinkedIn API enables you to use LinkedIn’s data and features in your web and mobile applications for your unique business needs.
LinkedIn publishes the following APIs.
- Consumer Solutions Platform: Covers most of the basic features familiar to us, including profiles, networking, posts, and comments.
- Marketing Developer Platform: A superset of consumer platforms with advanced features such as organization management.
- Compliance API: Same as marketing platform, but with data security features required by regulated industries.
- Talent Solutions: Recruitment-related features available only to some partners.
- learning solutions: This is a feature of the LinkedIn learning platform and is only available to select partners.
sales navigator: Features related to sales use cases, available to select partners only.
Get ready to use the LinkedIn API
The Consumer API provides most LinkedIn functionality without app authorization. However, in order to use features such as posting on company pages, apps must be approved by the marketing platform. This section describes the general steps to access both platforms.
1. App registration
- Log in to LinkedIn Developer Portal.
- Create a new app with “Create App”.
- Enter basic details like your name and logo.
- You need to associate your app with your company page. If the page does not exist, create one.
2. Request app verification on company page
- On the Settings tab, click “Confirm” to receive a verification link.
- Send that link to the admins of the previously selected company page.
- When an admin opens that link, they’re prompted to confirm their accountability for the app. When they confirm, you will receive a notification that the app has been verified and you can proceed with other settings.
3. Request access to features
- Request access to both “Share on LinkedIn” and “Sign in with LinkedIn” on the Products tab. They constitute a consumer platform.
- Request access to the “Marketing Developer Platform” to post on company pages. However, you must get approval after providing the details of your application.
- your application for a moment We review and anticipate a few rejections before being approved.
4. Application Approval Review
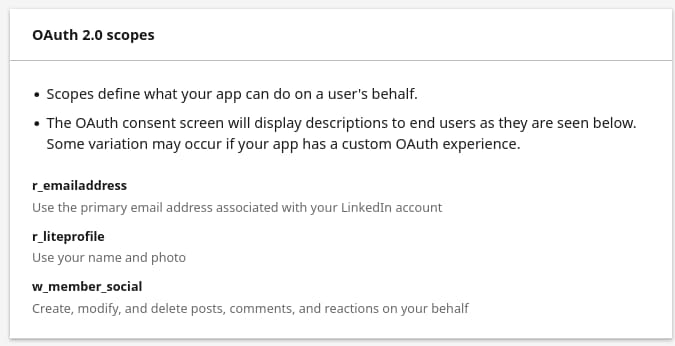
In the Authentication tab, register your app’s OAuth redirect URL. You will use it later to get the access token.
In the OAuth 2.0 Scopes section, verify that your app has the following scopes:
w_member_social
r_liteprofile
w_organization_social
(only if permission to access marketing platform is requested)
Store your client ID and client secret securely in a database or vault.
Get permission from user
The app must ask the user to allow them to operate their LinkedIn account. This is done using the OAuth 2.0 authorization code flow. After user authentication through LinkedIn and approval of the app, it generates an access token per user so that the application can make API calls to operate user accounts on its behalf.
after Authorization API Documentation We will not repeat the steps here as they are comprehensive and accurate. However, some details to keep in mind are:
- To write a post on your LinkedIn profile, you will need:
scope=r_liteprofile w_member_social
. - Add to Posts on Company Page
w_organization_social
to the range. - Access tokens do not expire for 60 days. Treat it like a password and store it safely in your user database.
Unlike many other OAuth services, LinkedIn does not issue refresh tokens to anyone. only part Approved Partner get them remember the refresh token if you get one Does not expire for 1 year.
What you need to know about the LinkedIn API
To avoid breaking client applications, LinkedIn maintains multiple versions of the API, each with different semantics. As of November 2022, three publishing APIs exist across different versions. Conflicting or incomplete information in documentation only adds to the confusion.
We’ve come up with the following tips to help you avoid the confusion by combing through the documentation.
- always use Posts API. it replaces both User Generated Content API and Shared API.
- that much Base URL Most REST APIs are now
/rest/
no/v2/
. For the Posts API, use:/rest/posts
no/v2/posts
. The latter still works but is deprecated. - An exception to the previous point is: Profile API. continue to use
/v2/me
no/rest/me
because the latter throwsACCESS_DENIED
error. - Several versions of the API exist, so you need to specify which one you want.
Linkedin-Version: yyyymm
header. The API then works as described in the documentation for that version.
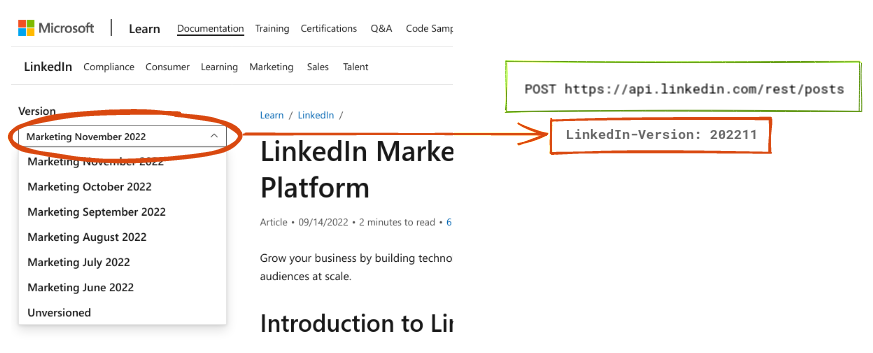
Once you’ve got those items sorted out, you’re ready to write your first post.
Create your first post (share) with the LinkedIn API
To share a post /post
LinkedIn POST endpoint:
POST https://api.linkedin.com/rest/posts
The body of the request is JSON object:
{
"author": "urn:li:person:{ID}",
"commentary": "Text of the post",
"visibility": "PUBLIC",
"lifecycleState": "PUBLISHED",
"distribution": {
"feedDistribution": "MAIN_FEED",
"targetEntities": [],
"thirdPartyDistributionChannels": []
}
}
author={urn}
: To post to user page uniform resource name (URN) “Likeurn:li:person:{ID}
Get that ID from “./v2/me
profile endpoint.
To post on a client’s company page, click “urn:li:organization:{ID}
(Note: This requires Marketing Platform authorization for the app and the Page Admin role for the user.)commentary={text}
: The text of the post.
Always add these 4 request headers:
Authorization: Bearer {access_token}
LinkedIn-Version: {yyyymm}
: API version that the application can handle.X-Restli-Protocol-Version: 2.0.0
Content-Type: application/json
If the request is successful, you will see a status of 201 (created) and x-restli-id
The response header contains the URN of the new post. urn:li:share:{ID}
.
Code using server-side Node.js with JavaScript:
import fetch from 'node-fetch';
// Get the access token for the current user.
// from your database or vault.
const accessToken = getAccessTokenOfLoggedInUser();
const headers = {
'Authorization': `Bearer ${accessToken}`,
'LinkedIn-Version': '202211',
'X-Restli-Protocol-Version': '2.0.0',
'Content-Type': 'application/json'
};
// Get user ID and cache it.
const apiResp = await fetch('https://api.linkedin.com/v2/me',
{headers: headers});
const profile = await apiResp.json();
const params = {
"author": `urn:li:person:${profile.id}`,
"commentary": "Text of the post",
"visibility": "PUBLIC",
"lifecycleState": "PUBLISHED",
"distribution": {
"feedDistribution": "MAIN_FEED",
"targetEntities": [],
"thirdPartyDistributionChannels": []
}
};
const postResp = await fetch(
'https://api.linkedin.com/rest/posts', {
method: 'POST',
headers: headers,
body: JSON.stringify(params)
});
console.log(postResp.status);
if (postResp.ok) {
const postId = postResp.headers.get('x-restli-id');
console.log(postId);
}
Get analytics for posts using the LinkedIn API
LinkedIn reports detailed metrics about company Pages and posts from those Pages. page statistics and shared stats end point. To receive analytics, your app must have LinkedIn Marketing Platform Authorization. Also, metrics are not available for posts on a user’s personal page.
Send this API request to get metrics for a post.
GET https://api.linkedin.com/rest/organizationalEntityShareStatistics?
organizationalEntity=urn:li:organization:{org-id}
&shares[0]=urn:li:share:{post-id}
organizationalEntity=urn:li:organization:{org-id}
: URN of your or customer company pageshares[0]=urn:li:share:{post-id}
: URN of the post on the page you want metrics for
The data in the response is:
{
"paging": {
"count": 3,
"start": 0
},
"elements": [
{
"totalShareStatistics": {
"uniqueImpressionsCount": 927,
"clickCount": 10976,
"engagement": 0.0095647t9487,
"likeCount": 31,
"commentCount": 23,
"shareCount": 2,
"commentMentionsCount": 2,
"impressionCount": 34416,
"shareMentionsCount": 2
},
"organizationalEntity": "urn:li:organization:151279"
}
]
}
A paging object is returned if the API returns a large number of objects. pagination. for example:
GET https://api.linkedin.com/v2/{service}?start=10&count=10
Alternative social media API for LinkedIn
Ayrshare Social Media API is a simpler alternative to LinkedIn API for posting and analyzing content.
Ready to use Ayrshare API
Get started with Ayrshare API in just 3 steps.
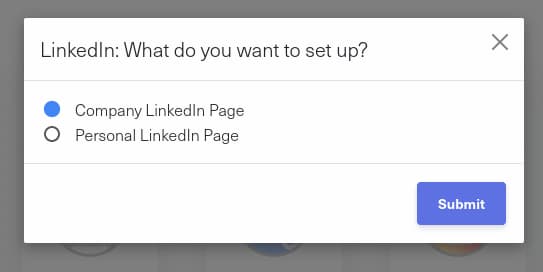
All set up to use the API as shown in the example below.
Publish videos from your organization to LinkedIn using Python
Would you like to automatically post to your or your company’s LinkedIn Page? For example, whenever a marketer uploads a helpful product training video, they’ll want it to appear on their company’s LinkedIn Page as well.
To do so, call Ayrshare. /post
endpoint video mediaUrls
Parameters (Note: You need a Premium or Business plan to post videos, but text posts and single image posts are available on all plans):
import os
import requests
# Add the API key to your application’s environment.
# export AYRSHARE_API_KEY=YOUR-AYRSHARE-API-KEY
params = {
'post': 'Product training video for our latest model WM300',
'mediaUrls': ['https://TRAINING-VIDEO-LINK'],
'platforms': ['linkedin']
}
headers = {
'Authorization': f'Bearer {os.environ["AYRSHARE_API_KEY"]}',
'Content-Type': 'application/json'
}
r = requests.post('https://app.ayrshare.com/api/post',
json=params, headers=headers)
ret = r.json()
You will receive a JSON response like this:
{
"status": "success",
"errors": [],
"postIds": [{
"status": "success",
"platform": "linkedin",
"postUrl": "https://www.linkedin.com/feed/update/urn:li:share:699863680",
"id": "urn:li:share:699863680"
}],
“id": "JsuoeTwnUSncf",
"post": "Product training video for our latest model WM300"
}
you can also use Social API PyPi Package to make the call simpler.
Post User Content on LinkedIn Company Pages
You can repost for any content (e.g. work) posted by users on your platform. their LinkedIn page. To do so, call the same /post
endpoint, but its user’s profile key at profileKeys
parameter.
This is shown in the Node.js snippet below (note: run server side, not browser. Do not store API key in browser).
import fetch from 'node-fetch'; // npm i node-fetch
// Add your API key to your application’s environment.
// export AYRSHARE_API_KEY=YOUR-AYRSHARE-API-KEY
const params = {
'post': 'Job description posted by your user on your platform...',
'platforms': ['linkedin'],
'profileKeys': ['PROFILE-KEY-OF-USER-FROM-YOUR-DATABASE']
}
const apiResp = await fetch('https://app.ayrshare.com/api/post', {
method: 'POST',
headers: {
'Authorization': `Bearer ${process.env.AYRSHARE_API_KEY}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(params)
});
const post = await apiResp.json();
you can also use Social API NPM Package to make the call simpler.
Get insight into your posts
using /analytics
Endpoints can get insight into how posts are performing and present them to users.
POST https://app.ayrshare.com/api/analytics/post
You will receive metrics such as:
...
"linkedin": {
"analytics": {
"uniqueImpressionsCount": 45, // Unique impressions for a post
"shareCount": 2, // Shares on a post
"engagement": 34, // Clicks, likes, comments, shares
"clickCount": 5, // Clicks on a post
"likeCount": 3, // Likes on a count
"impressionCount": 100, // Impressions on a post
"commentCount": 2 // Comments count
},
},
...
reference Analytics API more details
Extend your B2B marketing with the LinkedIn API
This article introduced you to more effective B2B marketing using the publishing capabilities of the LinkedIn API. For more information about LinkedIn, read our articles on the Best Social Media Platforms for B2B Customer Acquisition and Top 10 Social Media APIs for Developers.