Unless you’ve been living under a metaphorical tech rock, you’ve heard of (or tried) ChatGPT. As you can see, OpenAI is a very good chatbot. After launching in November 2022, ChatGPT is only available through the GUI interface. By “available” we mean the OpenAPI high-level language model “gpt-3.5-turbo” which supports ChatGPT. Other language models exist that aren’t that great. Recently, however, OpenAI announced its commitment. ChatGPT API You can call “gpt-3.5-turbo” via API. Dear friends, this opens up a world of new applications including social media posts created by ChatGPT.
Why use ChatGPT API for social?
There are several reasons you might want to integrate the ChatGPT API into your platform or app.
User content creation on the platform
Users create content such as images or videos on the platform and share it on social networks, but the friction point is writing social post content. For example, the Creators Marketplace creates stunning images of cats sitting on shoes. It’s promoting shoes, not cats. There is a button to share a picture of a shoe cat to Instagram.
But what text do you want to add to that post and what hashtag do you want to add? Do you let users fill it out, or use template text? Neither is a good solution.
ChatGPT is hot right now
Everyone is talking about ChatGPT, integrating AI into the platform is something to do, and some public companies are AI Integration Announcement. It may feel like you’re following the crowd, but it’s truly useful for you and your customers. If you can remove friction, increase customer satisfaction, and increase your bottom line, do it.
Generate social media post text using ChatGPT API
Let’s start the fun, create social media posts with text from ChatGPT API and post to Instagram and Facebook using Ayrshare’s social media API. we are AI generated image Use DALL E.
Get Chat API Key
the first step is Sign up for an OpenAI account Generate an API key. Just click on your profile icon in the top right corner and select “View API Key”.
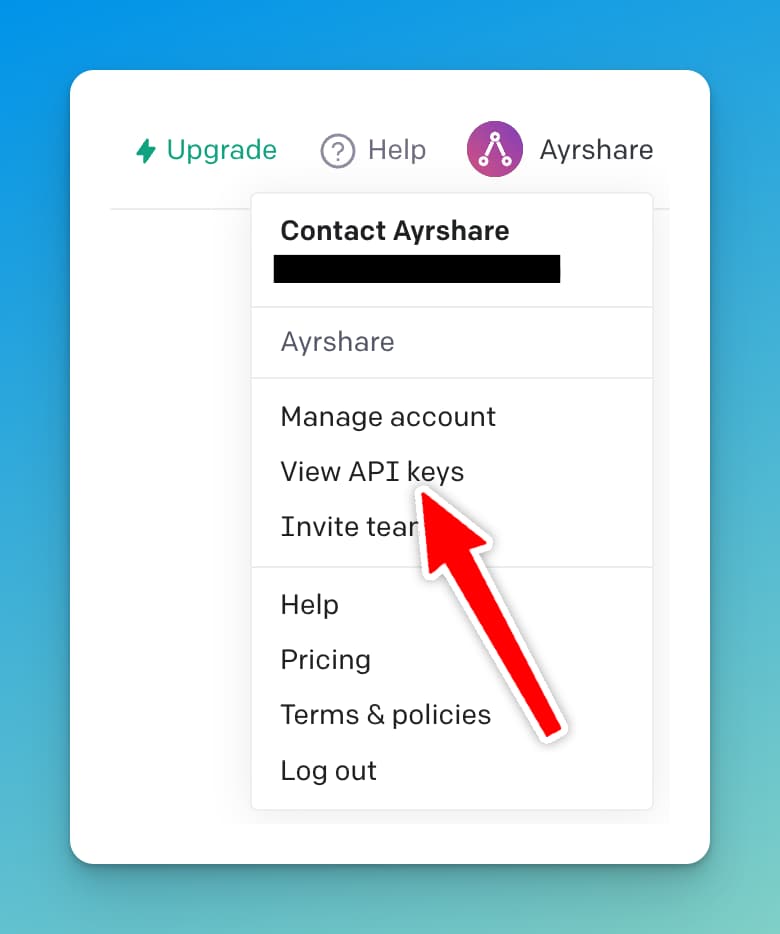
Signing up for Open AI is free and gives you plenty of credits to try things out.
Call the ChatGPT API endpoint
With your API key in hand, let’s make our first API endpoint call. All requests to ChatGPT should be something like “Please write a blog post about the popularity of Yellowstone TV shows.” This example uses the text “Write a social media post about a cat sitting on a shoe.” It also uses the GPT-3 language model. However, it is recommended to use the newer language model, GPT-4, as it gives better results.
Here is a cURL example.
curl https://api.openai.com/v1/chat/completions
-H "Authorization: Bearer $OPENAI_API_KEY"
-H "Content-Type: application/json"
-d '{
"model": "gpt-3.5-turbo",
"messages": [{"role": "user", "content": "What is the OpenAI mission?"}]
}'
We’ll use a JavaScript example going forward, but since it’s a REST call, you can write it in your favorite programming language like Python or PHP.
const OPENAI_API_KEY = "Your API OpenAI Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"https://api.openai.com/v1/chat/completions",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
};
run();
What does this code do? A detailed guide can be found here: OpenAI documentationBut here’s a quick summer.
- POST call to URL
https://api.openai.com/v1/chat/completions
For ChatGPT API requests. Authorization
: Include the OpenAI API Key in the header.model
: Write the model name in the body of the post. For ChatGPTgpt-3.5-turbo
.role
: any onesystem
,user
orassistant
. we want to useuser
Because we are asking for something.content
: A question or request to ChatGPT.
Invocation result:
{
"id": "chatcmpl-6ruxjLkNNtIKeZ0dPdCGG2ijXIYHd",
"object": "chat.completion",
"created": 1678308367,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 56,
"total_tokens": 75
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nnThis adorable little kitten has found a new favorite spot to lounge around in! Can you guess what it is? Yep, you got it- a shoe! How cute is this? 😍😻 #catinshoe #kittenlove #cutenessoverload"
},
"finish_reason": null,
"index": 0
}
]
}
ChatGPT Helper content
field:
nnThis adorable little kitten has found a new place to laze! Can you guess what it is? Yes, I see- shoes! How cute is this? 😍😻 #catinshoe #kittenlove #cutenessoverload
Not only is this a great summary, but it also has emojis and hashtags. Calling the endpoint again will give you different results.
post on social networks
Now that we have the text for the post, let’s post it. We will be using Ayrshare’s social API, you can sign up for a free account to test it out. Sign up and get your Ayrshare API key. The last key you need is the Social Accounts tab where you can link some of your social accounts like Facebook, Instagram and Twitter.
Now update the code to take the generated text and post it to the social network using the cat in the shoe image.
const OPENAI_API_KEY = "Your API OpenAI Key";
const AYRSHARE_API_KEY = "Your API Ayrshare Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"https://api.openai.com/v1/chat/completions",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
const optionsPost = {
method: "POST",
headers: {
Authorization: `Bearer ${AYRSHARE_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
post: chatGPTResults.choices[0].message.content,
platforms: ["facebook", "instagram"],
mediaUrls: ["https://img.ayrshare.com/012/cat-in-shoe.jpg"],
}),
};
const postResults = await fetch(
"https://app.ayrshare.com/api/post",
optionsPost
).then((res) => res.json());
console.log("Ayrshare says:", JSON.stringify(postResults, null, 2));
};
run();
Responses from ChatGPT and Ayrshare:
ChatGPT says: {
"id": "chatcmpl-6rvrDplRIwdiUURwXzdU3iS0KiYUo",
"object": "chat.completion",
"created": 1678311807,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 80,
"total_tokens": 99
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
},
"finish_reason": null,
"index": 0
}
]
}
Ayrshare says: {
"status": "success",
"errors": [],
"postIds": [
{
"status": "success",
"id": "738681876342836_1098688181078083",
"postUrl": "https://www.facebook.com/738681876342836_1098688181078083",
"platform": "facebook"
},
{
"status": "success",
"id": "17987746003883214",
"postUrl": "https://www.instagram.com/p/Cpix-vLtaBq/",
"usedQuota": 1,
"platform": "instagram"
}
],
"id": "KRRormDnpBTgfKYadpmn",
"refId": "d93ca2784c9bf7bf83ce0bf081d16c91598aec28",
"post": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
}
Here are the actual posts on Instagram and Facebook.
the new world of social
We saw how easy it is to create content and post to social using the API. This is just the beginning of what can’t be done when combining AI-generated content with automated social posting.
Check out these articles to learn more about how ChatGPT works or social media APIs:
Create social posts and rewrite posts
If you’re looking for an endpoint that handles both social post writing or post rewriting (social networks don’t like duplicate posts), try our new feature. Create a ChatGPT-based/API endpoint.
The API handles creation, formatting, adding hashtags and emojis, and ensuring that posts are of the required length (e.g. Twitter and 280 characters).